Librería Estandar
Contents
Librería Estandar#
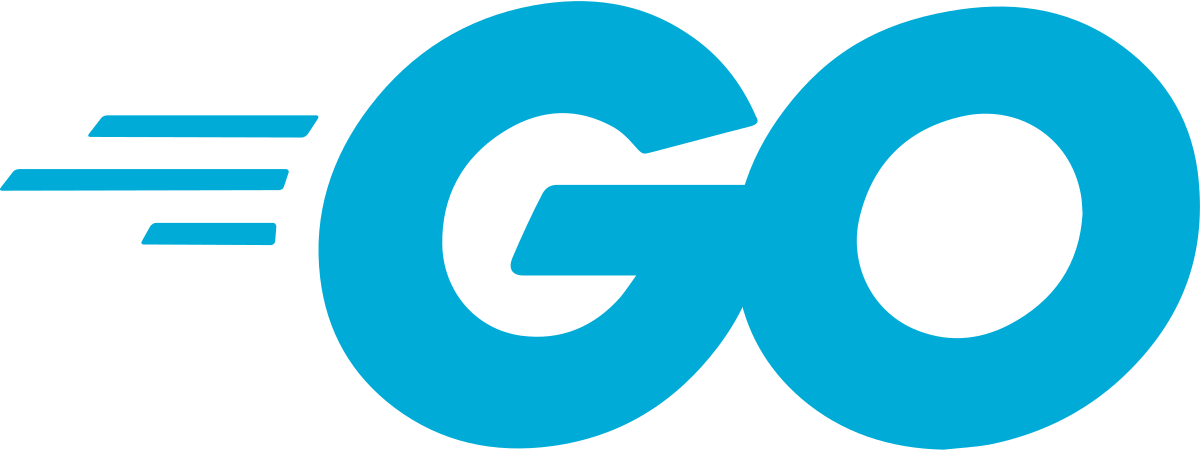
Paquetes más usados en GO
Índice
Manipulación del Sistema#
Averiguar el Sistema operativo (runtime)#
1package main
2
3// cargamos la librería runtime:
4import (
5 "fmt"
6 "runtime"
7)
8
9func main() {
10 // se averigua usando la variable runtime:
11 fmt.Println("Sistema operativo: ", runtime.GOOS)
12}
Averiguar la arquitectura#
1package main
2
3// cargamos la librería runtime:
4import (
5 "fmt"
6 "runtime"
7)
8
9func main() {
10 // se averigua usando la variable runtime:
11 fmt.Println("Arquitectura: ", runtime.GOARCH)
12}
Entrada y salida#
Impresión y recibir datos por consola#
1package main
2
3import (
4 "fmt" // importar fmt
5)
6
7func main() {
8 // imprimir con fmt:
9 fmt.Print("Hola persona.")
10 // imprimir con fmt y salto de línea:
11 fmt.Println("Hola persona y salto de línea.")
12
13 nombre := "Guillermo"
14 edad := 34
15
16 // Formatear información al imprimir:
17 fmt.Printf("Hola %s, tienes %v años. \n", nombre, edad) // con s se imprime variable string, con v se imprime algo que no sabemos su formato.
18
19 // Averiguar el tipo de dato de una variable:
20 fmt.Printf("%T \n", edad)
21
22 // recibir un dato:
23 fmt.Print("¿Cuál es tu nombre? >>> ")
24 fmt.Scanln(&nombre)
25
26 fmt.Println("Te llamas:", nombre)
27}
Nota
Al igual que print scan tiene las alternativas Scanln y Scanf que funcionan igual.
Cálculos y números#
Números aleatorios (math/rand)#
1package main
2
3// importar la librería rand:
4import (
5 "fmt"
6 "math/rand"
7)
8
9func main() {
10 // se establece el rango en la variable:
11 aleatorio := rand.Int()
12
13 fmt.Println("El número aleatorio es: ", aleatorio)
14}
Manipulación de datos#
Trabajar con strings (strings)#
1package main
2
3import (
4 "fmt"
5 "strings" // importar librería strings
6)
7
8// cargar la librería strings:
9
10func main() {
11 nombre := "Guillermo"
12
13 // conversión a mayúsculas:
14 fmt.Println(strings.ToUpper(nombre))
15
16 // conversión a minúsculas:
17 fmt.Println(strings.ToLower(nombre))
18
19 // reemplazar 1 o varios caracteres que encuentre:
20 nombre = strings.Replace(nombre, "ll", "y", 1)
21 fmt.Println(nombre)
22
23 // reemplazar todos los caracteres:
24 frase := "Mi-nombre-es-Guillermo"
25 frase = strings.ReplaceAll(frase, "-", " ")
26 fmt.Println(frase)
27
28 nombres := "Antonio, Luis, Alfredo, Pepa"
29
30 // Convertir a array, se crea una nueva variable para que cambie el tipo de dato a array:
31 listado := strings.Split(nombres, ", ")
32 fmt.Println(listado)
33
34 // Unir array en una cadena:
35 lista_cadena := strings.Join(listado, ", ")
36 fmt.Println(lista_cadena)
37
38}
Trabajar con Números (strconv)#
1package main
2
3import (
4 "fmt"
5 "strconv" // importar librería strings
6)
7
8func main() {
9 a := "20"
10 b := "30"
11 // convertir a entero sin variable de error:
12 a2, _ := strconv.Atoi(a)
13
14 // convertir con variable de error:
15 b2, err := strconv.Atoi(b)
16 // en este caso manejamos el error:
17 if err != nil {
18 fmt.Println(err)
19 fmt.Println("No se puede convertir el valor")
20 }
21
22 total := a2 + b2
23
24 fmt.Println(total)
25
26 // Otros tipos de conversión (manual de GO):
27 booleano, _ := strconv.ParseBool("true")
28 float, _ := strconv.ParseFloat("3.1415", 64)
29 integer, _ := strconv.ParseInt("-42", 10, 64)
30 uinteger, _ := strconv.ParseUint("42", 10, 64)
31
32 fmt.Println(booleano, float, integer, uinteger)
33
34}
Nota
Se puede convertir de entero a cadena usando la función Itoa() en lugar de Atoi()
Trabajar con errores (errors)#
1package main
2
3import (
4 "errors" // cargamos librería de errores
5 "fmt"
6)
7
8// la función retornará dos valores, un entero y un error:
9func calcular(num1, num2 int) (int, error) {
10 if num1 < num2 {
11 // es posible generar errores:
12 return 0, errors.New("No se aceptan valores negativos")
13 } else {
14 // hay que retornar dos cosas, si no ya error retornamos nil:
15 return num1 - num2, nil
16 }
17}
18
19func main() {
20 // recuperamos el resultado de la función en dos variables:
21 total, err := calcular(8, 16)
22
23 if err == nil {
24 fmt.Println("Total:", total)
25 } else {
26 fmt.Println(err)
27 }
28}
Manipulación de archivos#
Leer archivo (os)#
1package main
2
3import (
4 "fmt"
5 "log"
6 "os"
7)
8
9func main() {
10 // abrir un archivo nuevo:
11 file, err := os.ReadFile("archivo.txt")
12
13 // comprobar si existen errores:
14 if err != nil {
15 log.Fatal(err, "no es posible abrir el archivo")
16 }
17
18 // imprimir convirtiendo el contenido a string:
19 fmt.Println(string(file))
20}
Gestión del Tiempo#
Librería (time):
1package main
2
3import (
4 "fmt"
5 "time"
6)
7
8func main() {
9 // recuperar el momento actual:
10 inicio := time.Now()
11
12 for i := 0; i < 1000; i++ {
13 // mostrar tiempo pasado:
14 pasado := time.Since(inicio)
15 fmt.Println("Tiempo pasado:", pasado)
16 }
17
18}